Air Pollution Monitoring System using Arduino and MQ135 Air Quality Sensor
Hello friends! Welcome back to ElectroDuino. This blog is based on Air Pollution Monitoring System using Arduino and MQ135 Air Quality Sensor. Here we will discuss Introduction to Air Pollution Monitoring System, Project Concept, Block Diagram, Components Required, Circuit Diagram, Working Principle, and Arduino code.
Introduction
Day to day, the level of Air pollution is increasing rapidly due to increase industries, factories, vehicle use which affect human health. So here we have designed a device/system which can measure air quality around it and monitor air pollution levels and also indicates and warns us when the air quality goes down beyond a certain level. This system can sense NH3, NOx, alcohol, Benzene, smoke, CO2, and some other gases, these gases are harmful to human health. It has a small display that will show the air quality value in the PPM unit. So this system is perfect for Air Quality Monitoring. This is a small portable device, we can use it at our home, office, classroom, and factory. It can save us from harmful gases.
Project Concept
The key components of the Air Pollution Monitoring System are MQ135, Arduino board, OLED Display, LEDs, and buzzer. The MQ135 is one type of gas sensor that can sense NH3, NOx, alcohol, Benzene, smoke, CO2, and some other gases, these gases are harmful to human health. Arduino is the main microcontroller board of this system. The gas sensor continuously measures air quality and sends data to the Arduino board. Then Arduino prints air quality value on the OLED display in the PPM unit. The LEDs and Buzzer used as indicators, that indicates the air quality is in good, Poor, or dangerous zone.
Block Diagram of Air Pollution Monitoring System using Arduino and MQ135
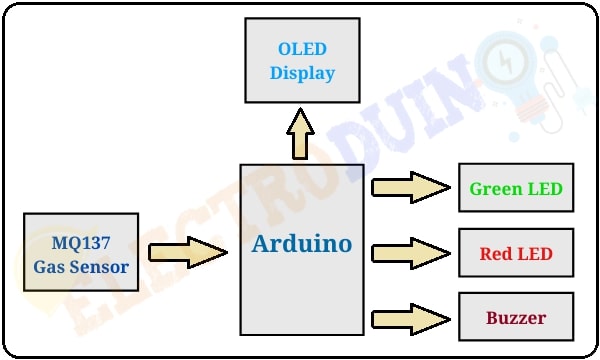
Components Required
Components Name | Quantity |
Arduino Nano | 1 |
MQ135 Gas Sensor | 1 |
0.96 inch I2C OLED display | 1 |
Green LED | 1 |
Red LED | 1 |
Buzzer | 1 |
220 ohm Resistor | 2 |
Slide Switch | 1 |
9V Battery with Battery connector | 1 |
PCB Zero board | 1 |
Connecting wires | As required in the circuit diagram |
LED or Light Emitting Diode – Pin Diagram, Construction, Working Principle |
Tools Required
Tools Name | Quantity |
Soldering Iron | 1 |
Soldering wire | 1 |
Soldering flux | 1 |
Soldering stand | 1 |
Multimeter | 1 |
Desoldering pump | 1 |
Wirecutter | 1 |
Required Library
We need to add 3 libraries in Arduino IDE software. These are:
- Download the MQ135.h library here: CLICK
- Download the Adafruit_GFX.h library here: CLICK
- Download the Adafruit_SSD1306.h library here: CLICK
Circuit Diagram of Air Pollution Monitoring System using Arduino and MQ135
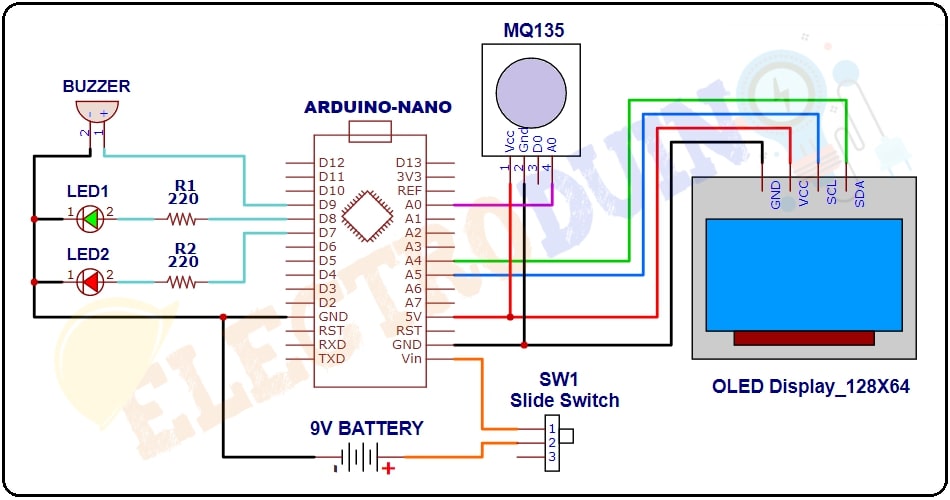
Working Principle of Air Pollution Monitoring System using Arduino and MQ135
When we turn on the system’s power supply, the MQ135 gas sensor starts sensing NH3, NOx, alcohol, Benzene, smoke, CO2, and some other harmful gases and it gives the output in form of voltage levels. This output voltage goes to the Arduino. Then the Arduino converts this voltage into PPM (parts per million) value with the help of the “MQ135.h” library which is defined in Arduino Code. Then the PPM value will print on the OLED display.
When no harmful gases are present around the sensor, it will give us a value of 90 PPM. Normally the safe level of air quality is 350 PPM and it should not exceed the quality level of 1000 PPM. When the air quality level exceeds 1000 PPM, then it starts to cause Headaches, sleepiness, and stagnant, stale. When this level exceeds 2000 ppm, it can cause increased heart rate and many other diseases.
For this reason, this system/device has indicators. When the air quality level is less than 1000 ppm, it indicates by turn on the green LED and the “Fresh Air” message is print on the Display. When this value will exceed 1000 PPM, it means the air is polluted, then the system indicates it by turn on the Red LED, and the “Poor Air” message is print on the Display. If this value will exceed 2000 PPM, it means the air is highly polluted, then the system indicates it by turn on the Red LED and Buzzer, and the “Danger! Air” message is print on the Display.
Arduino Code
/* Air Pollution Monitoring System using Arduino and MQ135 Air Quality Sensor www.Electroduino.com */ #include "MQ135.h" //OLED Display libraries #include <SPI.h> #include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h> #define SCREEN_WIDTH 128 // OLED display width, in pixels #define SCREEN_HEIGHT 64 // OLED display height, in pixels #define OLED_RESET 4 Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET); //In and Out int RedLed = 9; int GreenLed = 8; int Buzzer = 7; void setup() { pinMode(RedLed, OUTPUT); // initialize digital pin RedLed as an output. pinMode(GreenLed, OUTPUT); // initialize digital pin GreenLed as an output. pinMode(Buzzer, OUTPUT); // initialize digital pin Buzzer as an output. // Start serial communication between arduino and your computer Serial.begin(9600); //initialize with the I2C addr 0x3C (128x64) display.begin(SSD1306_SWITCHCAPVCC, 0x3C); display.clearDisplay(); delay(10); // Print text on display display.clearDisplay(); display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0,0); display.println("ELECTRODUIN0"); // Print text display.display(); display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0,20); display.println("Air Pollution"); display.display(); display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0,35); display.println(" Monitoring"); display.display(); delay(2000); } void loop() { // Read Senso value MQ135 gasSensor = MQ135(A0); float air_quality = gasSensor.getPPM(); // Print Senso value on Serial Monitor Window Serial.print("Air Quality: "); Serial.print(air_quality); Serial.println(" PPM"); Serial.println(); //Print Senso value or Air Quality Index on OLED Display display.clearDisplay(); display.setCursor(0,0); //oled display position display.setTextSize(1); display.setTextColor(WHITE); display.println("Air Quality Index"); display.setCursor(0,20); //oled display position display.setTextSize(2); display.setTextColor(WHITE); display.print(air_quality); display.setTextSize(1); display.setTextColor(WHITE); display.println(" PPM"); display.display(); // when air quality value less than 1000PPM if (air_quality<=1000) { digitalWrite(GreenLed, HIGH); // turn the Green LED on digitalWrite(RedLed,LOW); // turn the Red LED off noTone(Buzzer); // turn the Buzzer off // Print text on OLED Display display.setTextSize(2); display.setTextColor(WHITE); display.setCursor(0,45); display.println("Fresh Air"); //Message display.display(); delay(2000); } // when air quality value greater than 1000PPM & less than 2000PPM else if( air_quality>=1000 && air_quality<=2000 ) { digitalWrite(GreenLed,LOW); // turn the Green LED off digitalWrite(RedLed, HIGH ); // turn the Red LED on noTone(Buzzer); // turn the Buzzer off // Print text on OLED Display display.setTextSize(2); display.setTextColor(WHITE); display.setCursor(0,45); display.println(" Poor Air"); //Message display.display(); delay(2000); } // when air quality value greater than 2000PPM else if (air_quality>=2000 ) { digitalWrite(GreenLed,LOW); // turn the Green LED off digitalWrite(RedLed,HIGH); // turn the Red LED on tone(Buzzer, 1000, 200); // turn the Buzzer on // Print text on OLED Display display.setTextSize(2); display.setTextColor(WHITE); display.setCursor(0,45); display.println("Danger!!"); //Message display.display(); delay(2000); } }